Each element in the list is represented by a node, the level of the node is chosen randomly while insertion in the list. **Level does not depend on the number of elements in the node.
randomLevel()
lvl := 1
//random() that returns a random value in [0...1)
while random() < p and lvl < MaxLevel do
lvl := lvl + 1
return lvl
Following is the pseudo-code for the insertion algorithm
**Insert(list, searchKey)**
local update[0...MaxLevel+1]
x := list -> header
for i := list -> level downto 0 do
while x -> forward[i] -> key forward[i]
update[i] := x
x := x -> forward[0]
lvl := randomLevel()
if lvl > list -> level then
for i := list -> level + 1 to lvl do
update[i] := list -> header
list -> level := lvl
x := makeNode(lvl, searchKey, value)
for i := 0 to level do
x -> forward[i] := update[i] -> forward[i]
update[i] -> forward[i] := x
// C++ code for inserting element in skip list
#include <bits/stdc++.h>
using namespace std;
// Class to implement node
class Node
{
public:
int key;
// Array to hold pointers to node of different level
Node **forward;
Node(int, int);
};
...
Node::Node(int key, int level)
{
this->key = key;
// Allocate memory to forward
forward = new Node*[level+1];
// Fill forward array with 0(NULL)
memset(forward, 0, sizeof(Node*)*(level+1));
};
...
// Class for Skip list
class SkipList
{
// Maximum level for this skip list
int MAXLVL;
// P is the fraction of the nodes with level
// i pointers also having level i+1 pointers
float P;
// current level of skip list
int level;
// pointer to header node
Node *header;
public:
SkipList(int, float);
int randomLevel();
Node* createNode(int, int);
void insertElement(int);
void displayList();
};
...
SkipList::SkipList(int MAXLVL, float P)
{
this->MAXLVL = MAXLVL;
this->P = P;
level = 0;
// create header node and initialize key to -1
header = new Node(-1, MAXLVL);
};
...
// create random level for node
int SkipList::randomLevel()
{
float r = (float)rand()/RAND_MAX;
int lvl = 0;
while (r < P && lvl < MAXLVL)
{
lvl++;
r = (float)rand()/RAND_MAX;
}
return lvl;
};
...
// create new node
Node* SkipList::createNode(int key, int level)
{
Node *n = new Node(key, level);
return n;
};
...
// Insert given key in skip list
void SkipList::insertElement(int key)
{
Node *current = header;
// create update array and initialize it
Node *update[MAXLVL+1];
memset(update, 0, sizeof(Node*)*(MAXLVL+1));
/* start from highest level of skip list
move the current pointer forward while key
is greater than key of node next to current
Otherwise inserted current in update and
move one level down and continue search
*/
for (int i = level; i >= 0; i--)
{
while (current->forward[i] != NULL &&
current->forward[i]->key < key)
current = current->forward[i];
update[i] = current;
}
/* reached level 0 and forward pointer to
right, which is desired position to
insert key.
*/
current = current->forward[0];
...
...
/* if current is NULL that means we have reached
to end of the level or current's key is not equal
to key to insert that means we have to insert
node between update[0] and current node */
if (current == NULL || current->key != key)
{
// Generate a random level for node
int rlevel = randomLevel();
// If random level is greater than list's current
// level (node with highest level inserted in
// list so far), initialize update value with pointer
// to header for further use
if (rlevel > level)
{
for (int i=level+1;i<rlevel+1;i++)
update[i] = header;
// Update the list current level
level = rlevel;
}
// create new node with random level generated
Node* n = createNode(key, rlevel);
// insert node by rearranging pointers
for (int i=0;i<=rlevel;i++)
{
n->forward[i] = update[i]->forward[i];
update[i]->forward[i] = n;
}
cout << "Successfully Inserted key " << key << "\n";
}
};
...
// Display skip list level wise
void SkipList::displayList()
{
cout<<"\n*****Skip List*****"<<"\n";
for (int i=0;i<=level;i++)
{
Node *node = header->forward[i];
cout << "Level " << i << ": ";
while (node != NULL)
{
cout << node->key<<" ";
node = node->forward[i];
}
cout << "\n";
}
};
...
// Driver to test above code
int main()
{
// Seed random number generator
srand((unsigned)time(0));
// create SkipList object with MAXLVL and P
SkipList lst(3, 0.5);
lst.insertElement(3);
lst.insertElement(6);
lst.insertElement(7);
lst.insertElement(9);
lst.insertElement(12);
lst.insertElement(19);
lst.insertElement(17);
lst.insertElement(26);
lst.insertElement(21);
lst.insertElement(25);
lst.displayList();
}
// Java code for inserting element in skip list
class GFG {
// Class to implement node
static class Node {
int key;
// Array to hold pointers to node of different level
Node forward[];
Node(int key, int level)
{
this.key = key;
// Allocate memory to forward
forward = new Node[level + 1];
}
};
...
// Class for Skip list
static class SkipList {
// Maximum level for this skip list
int MAXLVL;
// P is the fraction of the nodes with level
// i pointers also having level i+1 pointers
float P;
// current level of skip list
int level;
// pointer to header node
Node header;
...
SkipList(int MAXLVL, float P)
{
this.MAXLVL = MAXLVL;
this.P = P;
level = 0;
// create header node and initialize key to -1
header = new Node(-1, MAXLVL);
}
...
int randomLevel()
{
float r = (float)Math.random();
int lvl = 0;
while (r < P && lvl < MAXLVL) {
lvl++;
r = (float)Math.random();
}
return lvl;
}
...
Node createNode(int key, int level)
{
Node n = new Node(key, level);
return n;
}
...
// Insert given key in skip list
void insertElement(int key)
{
Node current = header;
// create update array and initialize it
Node update[] = new Node[MAXLVL + 1];
/* start from highest level of skip list
move the current pointer forward while
key is greater than key of node next to
current Otherwise inserted current in update
and move one level down and continue search
*/
for (int i = level; i >= 0; i--) {
while (current.forward[i] != null
&& current.forward[i].key < key)
current = current.forward[i];
update[i] = current;
}
/* reached level 0 and forward pointer to
right, which is desired position to
insert key.
*/
current = current.forward[0];
...
...
/* if current is NULL that means we have reached
to end of the level or current's key is not
equal to key to insert that means we have to
insert node between update[0] and current node
*/
if (current == null || current.key != key) {
// Generate a random level for node
int rlevel = randomLevel();
// If random level is greater than list's
// current level (node with highest level
// inserted in list so far), initialize
// update value with pointer to header for
// further use
if (rlevel > level) {
for (int i = level + 1; i < rlevel + 1;
i++)
update[i] = header;
// Update the list current level
level = rlevel;
}
// create new node with random level
// generated
Node n = createNode(key, rlevel);
// insert node by rearranging pointers
for (int i = 0; i <= rlevel; i++) {
n.forward[i] = update[i].forward[i];
update[i].forward[i] = n;
}
System.out.println(
"Successfully Inserted key " + key);
}
}
...
// Display skip list level wise
void displayList()
{
System.out.println("\n*****Skip List*****");
for (int i = 0; i <= level; i++) {
Node node = header.forward[i];
System.out.print("Level " + i + ": ");
while (node != null) {
System.out.print(node.key + " ");
node = node.forward[i];
}
System.out.println();
}
}
}
...
// Driver to test above code
public static void main(String[] args)
{
// create SkipList object with MAXLVL and P
SkipList lst = new SkipList(3, 0.5f);
lst.insertElement(3);
lst.insertElement(6);
lst.insertElement(7);
lst.insertElement(9);
lst.insertElement(12);
lst.insertElement(19);
lst.insertElement(17);
lst.insertElement(26);
lst.insertElement(21);
lst.insertElement(25);
lst.displayList();
}
}
// This code is contributed by Lovely Jain
Output
Successfully Inserted key 3
Successfully Inserted key 6
Successfully Inserted key 7
Successfully Inserted key 9
Successfully Inserted key 12
Successfully Inserted key 19
Successfully Inserted key 17
Successfully Inserted key 26
Successfully Inserted key 21
Successfully Inserted key 25
*****Skip List*****
Level 0: 3 6 7 9 12 17 19 21 25 26
Level 1: 3 6 12 17 25 26
Level 2: 3 6 12 25
Level 3: 3 25
Note: The level of nodes is decided randomly, so output may differ.
**Search(list, searchKey)**
x := list -> header
-- loop invariant: x -> key level downto 0 do
while x -> forward[i] -> key forward[i]
x := x -> forward[0]
if x -> key = searchKey then return x -> value
else return failure
**Delete(list, searchKey)**
local update[0..MaxLevel+1]
x := list -> header
for i := list -> level downto 0 do
while x -> forward[i] -> key forward[i]
update[i] := x
x := x -> forward[0]
if x -> key = searchKey then
for i := 0 to list -> level do
if update[i] -> forward[i] ≠ x then break
update[i] -> forward[i] := x -> forward[i]
free(x)
while list -> level > 0 and list -> header -> forward[list -> level] = NIL do
list -> level := list -> level – 1
// C++ code for searching and deleting element in skip list
#include <bits/stdc++.h>
using namespace std;
// Class to implement node
class Node
{
public:
int key;
// Array to hold pointers to node of different level
Node **forward;
Node(int, int);
};
...
Node::Node(int key, int level)
{
this->key = key;
// Allocate memory to forward
forward = new Node*[level+1];
// Fill forward array with 0(NULL)
memset(forward, 0, sizeof(Node*)*(level+1));
};
...
// Class for Skip list
class SkipList
{
// Maximum level for this skip list
int MAXLVL;
// P is the fraction of the nodes with level
// i pointers also having level i+1 pointers
float P;
// current level of skip list
int level;
// pointer to header node
Node *header;
...
...
public:
SkipList(int, float);
int randomLevel();
Node* createNode(int, int);
void insertElement(int);
void deleteElement(int);
void searchElement(int);
void displayList();
};
...
SkipList::SkipList(int MAXLVL, float P)
{
this->MAXLVL = MAXLVL;
this->P = P;
level = 0;
// create header node and initialize key to -1
header = new Node(-1, MAXLVL);
};
...
// create random level for node
int SkipList::randomLevel()
{
float r = (float)rand()/RAND_MAX;
int lvl = 0;
while(r < P && lvl < MAXLVL)
{
lvl++;
r = (float)rand()/RAND_MAX;
}
return lvl;
};
...
// create new node
Node* SkipList::createNode(int key, int level)
{
Node *n = new Node(key, level);
return n;
};
...
// Insert given key in skip list
void SkipList::insertElement(int key)
{
Node *current = header;
// create update array and initialize it
Node *update[MAXLVL+1];
memset(update, 0, sizeof(Node*)*(MAXLVL+1));
/* start from highest level of skip list
move the current pointer forward while key
is greater than key of node next to current
Otherwise inserted current in update and
move one level down and continue search
*/
for(int i = level; i >= 0; i--)
{
while(current->forward[i] != NULL &&
current->forward[i]->key < key)
current = current->forward[i];
update[i] = current;
}
...
...
/* reached level 0 and forward pointer to
right, which is desired position to
insert key.
*/
current = current->forward[0];
/* if current is NULL that means we have reached
to end of the level or current's key is not equal
to key to insert that means we have to insert
node between update[0] and current node */
if (current == NULL || current->key != key)
{
// Generate a random level for node
int rlevel = randomLevel();
/* If random level is greater than list's current
level (node with highest level inserted in
list so far), initialize update value with pointer
to header for further use */
if(rlevel > level)
{
for(int i=level+1;i<rlevel+1;i++)
update[i] = header;
// Update the list current level
level = rlevel;
}
// create new node with random level generated
Node* n = createNode(key, rlevel);
// insert node by rearranging pointers
for(int i=0;i<=rlevel;i++)
{
n->forward[i] = update[i]->forward[i];
update[i]->forward[i] = n;
}
cout<<"Successfully Inserted key "<<key<<"\n";
}
};
...
// Delete element from skip list
void SkipList::deleteElement(int key)
{
Node *current = header;
// create update array and initialize it
Node *update[MAXLVL+1];
memset(update, 0, sizeof(Node*)*(MAXLVL+1));
/* start from highest level of skip list
move the current pointer forward while key
is greater than key of node next to current
Otherwise inserted current in update and
move one level down and continue search
*/
for(int i = level; i >= 0; i--)
{
while(current->forward[i] != NULL &&
current->forward[i]->key < key)
current = current->forward[i];
update[i] = current;
}
...
...
/* reached level 0 and forward pointer to
right, which is possibly our desired node.*/
current = current->forward[0];
// If current node is target node
if(current != NULL and current->key == key)
{
/* start from lowest level and rearrange
pointers just like we do in singly linked list
to remove target node */
for(int i=0;i<=level;i++)
{
/* If at level i, next node is not target
node, break the loop, no need to move
further level */
if(update[i]->forward[i] != current)
break;
update[i]->forward[i] = current->forward[i];
}
// Remove levels having no elements
while(level>0 &&
header->forward[level] == 0)
level--;
cout<<"Successfully deleted key "<<key<<"\n";
}
};
...
// Search for element in skip list
void SkipList::searchElement(int key)
{
Node *current = header;
/* start from highest level of skip list
move the current pointer forward while key
is greater than key of node next to current
Otherwise inserted current in update and
move one level down and continue search
*/
for(int i = level; i >= 0; i--)
{
while(current->forward[i] &&
current->forward[i]->key < key)
current = current->forward[i];
}
/* reached level 0 and advance pointer to
right, which is possibly our desired node*/
current = current->forward[0];
// If current node have key equal to
// search key, we have found our target node
if(current and current->key == key)
cout<<"Found key: "<<key<<"\n";
};
...
// Display skip list level wise
void SkipList::displayList()
{
cout<<"\n*****Skip List*****"<<"\n";
for(int i=0;i<=level;i++)
{
Node *node = header->forward[i];
cout<<"Level "<<i<<": ";
while(node != NULL)
{
cout<<node->key<<" ";
node = node->forward[i];
}
cout<<"\n";
}
};
...
// Driver to test above code
int main()
{
// Seed random number generator
srand((unsigned)time(0));
// create SkipList object with MAXLVL and P
SkipList lst(3, 0.5);
lst.insertElement(3);
lst.insertElement(6);
lst.insertElement(7);
lst.insertElement(9);
lst.insertElement(12);
lst.insertElement(19);
lst.insertElement(17);
lst.insertElement(26);
lst.insertElement(21);
lst.insertElement(25);
lst.displayList();
//Search for node 19
lst.searchElement(19);
//Delete node 19
lst.deleteElement(19);
lst.displayList();
}
Successfully Inserted key 3
Successfully Inserted key 6
Successfully Inserted key 7
Successfully Inserted key 9
Successfully Inserted key 12
Successfully Inserted key 19
Successfully Inserted key 17
Successfully Inserted key 26
Successfully Inserted key 21
Successfully Inserted key 25
*****Skip List*****
Level 0: 3 6 7 9 12 17 19 21 25 26
Level 1: 6 9 19 26
Level 2: 19
Found key: 19
Successfully deleted key 19
*****Skip List*****
Level 0: 3 6 7 9 12 17 21 25 26
Level 1: 6 9 26
ip[] = {10, 5, 30, 40, 2, 4, 9}
op[] = {2, 4, 5, 9, 10, 30, 40}
ip[] = {1, 10, 7}
op[] = {1, 7, 10}
Let, input[] = {10, 5, 30, 40, 2, 4, 9}
Initialize: output[] = {}, sublist[] = {}
sublist[] = {10}
sublist[] = {10, 30, 40}, input[] = {5, 2, 4, 9}
op = {10, 30, 40}
sublist[] = {5}
input[] = {2, 4}
sublist[] = {5, 9}
output = {5, 9, 10, 30, 40}
{2, 4}
are first moved to sublist and then merged into output.output = {2, 4, 5, 9, 10, 30, 40}
ip[]
be input list and op[]
be output list.ip[]
to it.x
, check if x
is greater than last inserted item to sublist. If yes, remove x
from ip[]
and add at the end of sublist. If no, ignore x
(Keep it, it in ip[]
)op[]
(output list)ip[]
and current items in op[]
.// CPP program to implement Strand Sort
#include <bits/stdc++.h>
using namespace std;
// A recursive function to implement Strand
// sort.
// ip is input list of items (unsorted).
// op is output list of items (sorted)
void strandSort(list<int> &ip, list<int> &op)
{
// Base case : input is empty
if (ip.empty())
return;
// Create a sorted sublist with
// first item of input list as
// first item of the sublist
list<int> sublist;
sublist.push_back(ip.front());
ip.pop_front();
...
...
// Traverse remaining items of ip list
for (auto it = ip.begin(); it != ip.end(); ) {
// If current item of input list
// is greater than last added item
// to sublist, move current item
// to sublist as sorted order is
// maintained.
if (*it > sublist.back()) {
sublist.push_back(*it);
// erase() on list removes an
// item and returns iterator to
// next of removed item.
it = ip.erase(it);
}
// Otherwise ignore current element
else
it++;
}
...
...
// Merge current sublist into output
op.merge(sublist);
...
// Recur for remaining items in
// input and current items in op.
strandSort(ip, op);
} //end of function...
// Driver code
int main(void)
{
list<int> ip{10, 5, 30, 40, 2, 4, 9};
// To store sorted output list
list<int> op;
// Sorting the list
strandSort(ip, op);
// Printing the sorted list
for (auto x : op)
cout << x << " ";
return 0;
}
Sorting algorithms/Strand sort - Rosetta Code
#include <stdio.h>
typedef struct node_t *node, node_t;
struct node_t { int v; node next; };
typedef struct { node head, tail; } slist;
...
void push(slist *l, node e) {
if (!l->head) l->head = e;
if (l->tail) l->tail->next = e;
l->tail = e;
}
...
node removehead(slist *l) {
node e = l->head;
if (e) {
l->head = e->next;
e->next = 0;
}
return e;
}
...
void join(slist *a, slist *b) {
push(a, b->head);
a->tail = b->tail;
}
...
void merge(slist *a, slist *b) {
slist r = {0};
while (a->head && b->head)
push(&r, removehead(a->head->v <= b->head->v ? a : b));
join(&r, a->head ? a : b);
*a = r;
b->head = b->tail = 0;
}
...
void sort(int *ar, int len)
{
node_t all[len];
// array to list
for (int i = 0; i < len; i++)
all[i].v = ar[i], all[i].next = i < len - 1 ? all + i + 1 : 0;
slist list = {all, all + len - 1}, rem, strand = {0}, res = {0};
for (node e = 0; list.head; list = rem) {
rem.head = rem.tail = 0;
while ((e = removehead(&list)))
push((!strand.head || e->v >= strand.tail->v) ? &strand : &rem, e);
merge(&res, &strand);
}
// list to array
for (int i = 0; res.head; i++, res.head = res.head->next)
ar[i] = res.head->v;
}
...
void show(const char *title, int *x, int len)
{
printf("%s ", title);
for (int i = 0; i < len; i++)
printf("%3d ", x[i]);
putchar('\n');
}
...
# define SIZE sizeof(x)/sizeof(int)
int main(void)
{
int x[] = {-2,0,-2,5,5,3,-1,-3,5,5,0,2,-4,4,2};
show("before sort:", x, SIZE);
sort(x, sizeof(x)/sizeof(int));
show("after sort: ", x, SIZE);
return 0;
}
An array is a variable which can store multiple values of same data type at a time.
An array can also be defined as follows...
"Collection of similar data items stored in continuous memory locations with single name".
To understand the concept of arrays, consider the following example declaration.
int a, b, c
int a[3];
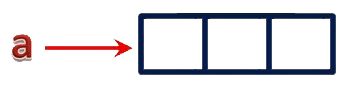
Index
".Index
values for the above example are as follows...name
and index
as follows...
arrayName[indexValue]
If I want to assign a value to any of these memory locations (array elements), we can assign as follows...
- a[1] = 100
;
The result will be as follows...
datatype arrayName [ size ] ;
int rollNumbers [60] ;
The above declaration of single dimensional array reserves 60 continuous memory locations of 2 bytes each with the name rollNumbers and tells the compiler to allow only integer values into those memory locations.
datatype arrayName [ size ] = {value1, value2, ...} ;
int marks [6] = { 89, 90, 76, 78, 98, 86 } ;
89
in first memory location, 90
in second memory location, 76
in third memory location, 78
in fourth memory location, 98
in fifth memory location and 86
in sixth memory location.datatype arrayName [ ] = {value1, value2, ...} ;
The array must be initialized if it is created without specifying any size. In this case, the size of the array is decided based on the number of values initialized
int marks [] = { 89, 90, 76, 78, 98, 86 } ;
char studentName [] = "btechsmartclass";
marks
is 6
and the size of the array studentName
is 16
.\0
(NULL)
at the end.Index
value of an element in an array is the reference number given to each element at the time of memory allocation.subscript
or indices
.arrayName [ indexValue ]
marks [2] = 99 ;
datatype arrayName [ rowSize ] [ columnSize ] ;
int matrix_A [2][3] ;
datatype arrayName [rows][colmns] = {
{r1c1value, r1c2value, ...},
{r2c1,r2c2,...}
...} ;
int matrix_A [2][3] = { {1, 2, 3},{4, 5, 6} } ;
We can also initialize as follows...
int matrix_A [2][3] = {
{1, 2, 3},
{4, 5, 6}
} ;
arrayName [ rowIndex ] [ columnIndex ]
matrix_A [0][1] = 10 ;
An example problem :
Suppose n people are sitting at a circular table with names A, B, C, D, ... Given a name, we need to print all n people (in order) starting from the given name.
For example, consider people and given name as . People sitting in a circular manner starting from are .
A simple solution is to create an auxiliary array of size and store it in another array. For example for people, we create below the auxiliary array.
Now for any given index, we simply print n elements starting from it. For example, we print the following .
A B C D E F A B C D E F
Below is the implementation of the above approach.
// CPP program to demonstrate use of circular
// array using extra memory space
#include <bits/stdc++.h>
using namespace std;
void print(char a[], int n, int ind)
{
// Create an auxiliary array of twice size.
char b[(2 * n)];
// Copy a[] to b[] two times
for (int i = 0; i < n; i++)
b[i] = b[n + i] = a[i];
// print from ind-th index to (n+i)th index.
for (int i = ind; i < n + ind; i++)
cout << b[i] << " ";
}
// Driver code
int main()
{
char a[] = { 'A', 'B', 'C', 'D', 'E', 'F' };
int n = sizeof(a) / sizeof(a[0]);
print(a, n, 3);
return 0;
}
// Java program to demonstrate use of circular
// array using extra memory space
import java.util.*;
import java.lang.*;
public class GfG{
public static void print(char a[], int n,
int ind){
// Create an auxiliary array
// of twice size.
char[] b = new char[(2 * n)];
// Copy a[] to b[] two times
for (int i = 0; i < n; i++)
b[i] = b[n + i] = a[i];
// print from ind-th index to
// (n+i)th index.
for (int i = ind; i < n + ind; i++)
System.out.print(b[i]+" ");
}
...
...
// Driver code
public static void main(String argc[]){
char[] a = new char[]{ 'A', 'B', 'C',
'D', 'E', 'F' };
int n = 6;
print(a, n, 3);
}
}
/* This code is contributed by Sagar Shukla */
// C# program to demonstrate use of circular
// array using extra memory space
using System;
public class GfG {
public static void print(char[] a, int n,
int ind)
{
// Create an auxiliary array
// of twice size.
char[] b = new char[(2 * n)];
// Copy a[] to b[] two times
for (int i = 0; i < n; i++)
b[i] = b[n + i] = a[i];
// print from ind-th index to
// (n+i)th index.
for (int i = ind; i < n + ind; i++)
Console.Write(b[i] + " ");
}
...
// Driver code
public static void Main()
{
char[] a = new char[] { 'A', 'B', 'C',
'D', 'E', 'F' };
int n = 6;
print(a, n, 3);
}
}
/* This code is contributed by vt_m*/
arr[]
of size N
and an integer, the task is to rotate the array elements to the left
by d
positions.Input
arr[] = {1, 2, 3, 4, 5, 6, 7}
,d = 2
Output
3 4 5 6 7 1 2
Input:
arr[] = {3, 4, 5, 6, 7, 1, 2}
, d=2
Output:
5 6 7 1 2 3 4
Approach 1 (Using temp array)
Follow the steps below to solve the given problem.
#include <bits/stdc++.h>
using namespace std;
// Fuction to rotate array
void Rotate(int arr[], int d, int n)
{
// Storing rotated version of array
int temp[n];
// Keepig track of the current index
// of temp[]
int k = 0;
// Storing the n - d elements of
// array arr[] to the front of temp[]
for (int i = d; i < n; i++) {
temp[k] = arr[i];
k++;
}
// Storing the first d elements of array arr[]
// into temp
for (int i = 0; i < d; i++) {
temp[k] = arr[i];
k++;
}
// Copying the elements of temp[] in arr[]
// to get the final rotated array
for (int i = 0; i < n; i++) {
arr[i] = temp[i];
}
}
...
// Function to print elements of array
void PrintTheArray(int arr[], int n)
{
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
}
...
// Driver code
int main()
{
int arr[] = { 1, 2, 3, 4, 5, 6, 7 };
int N = sizeof(arr) / sizeof(arr[0]);
int d = 2;
// Function calling
Rotate(arr, d, N);
PrintTheArray(arr, N);
return 0;
}
/*package whatever //do not write package name here */
import java.io.*;
class GFG {
// Fuction to rotate array
static void Rotate(int arr[], int d, int n)
{
// Storing rotated version of array
int temp[] = new int[n];
// Keepig track of the current index
// of temp[]
int k = 0;
// Storing the n - d elements of
// array arr[] to the front of temp[]
for (int i = d; i < n; i++) {
temp[k] = arr[i];
k++;
}
// Storing the first d elements of array arr[]
// into temp
for (int i = 0; i < d; i++) {
temp[k] = arr[i];
k++;
}
// Copying the elements of temp[] in arr[]
// to get the final rotated array
for (int i = 0; i < n; i++) {
arr[i] = temp[i];
}
}
...
// Function to print elements of array
static void PrintTheArray(int arr[], int n)
{
for (int i = 0; i < n; i++) {
System.out.print(arr[i]+" ");
}
}
public static void main (String[] args) {
int arr[] = { 1, 2, 3, 4, 5, 6, 7 };
int N = arr.length;
int d = 2;
// Function calling
Rotate(arr, d, N);
PrintTheArray(arr, N);
}
}
// Include namespace system
using System;
public class GFG
{
// Fuction to rotate array
public static void Rotate(int[] arr, int d, int n)
{
// Storing rotated version of array
int[] temp = new int[n];
// Keepig track of the current index
// of temp[]
var k = 0;
// Storing the n - d elements of
// array arr[] to the front of temp[]
for (int i = d; i < n; i++)
{
temp[k] = arr[i];
k++;
}
// Storing the first d elements of array arr[]
// into temp
for (int i = 0; i < d; i++)
{
temp[k] = arr[i];
k++;
}
// Copying the elements of temp[] in arr[]
// to get the final rotated array
for (int i = 0; i < n; i++)
{
arr[i] = temp[i];
}
}
...
// Function to print elements of array
public static void PrintTheArray(int[] arr, int n)
{
for (int i = 0; i < n; i++)
{
Console.Write(arr[i].ToString() + " ");
}
}
...
public static void Main(String[] args)
{
int[] arr = {1, 2, 3, 4, 5, 6, 7};
var N = arr.Length;
var d = 2;
// Function calling
GFG.Rotate(arr, d, N);
GFG.PrintTheArray(arr, N);
}
}
arr[i] = i
N
, ranging from 0
to N – 1
.-1
present in the array.A[i] = i
and if i
is not present,
-1
at that place.arr = {-1, -1, 6, 1, 9, 3, 2, -1, 4, -1}
[-1, 1, 2, 3, 4, -1, 6, -1, -1, 9]
arr = {19, 7, 0, 3, 18, 15, 12, 6, 1, 8, 11, 10, 9, 5, 13, 16, 2, 14, 17, 4}
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19]
// C program for above approach
#include <stdio.h>
// Function to transform the array
void fixArray(int ar[], int n)
{
int i, j, temp;
// Iterate over the array
for (i = 0; i < n; i++)
{
for (j = 0; j < n; j++)
{
// Check is any ar[j]
// exists such that
// ar[j] is equal to i
if (ar[j] == i) {
temp = ar[j];
ar[j] = ar[i];
ar[i] = temp;
break;
}
}
}
...
...
// Iterate over array
for (i = 0; i < n; i++)
{
// If not present
if (ar[i] != i)
{
ar[i] = -1;
}
}
...
...
// Print the output
printf("Array after Rearranging\n");
for (i = 0; i < n; i++) {
printf("%d ",ar[i]);
}
}
...
// Driver Code
int main()
{
int n, ar[] = { -1, -1, 6, 1, 9, 3, 2, -1, 4, -1 };
n = sizeof(ar) / sizeof(ar[0]);
// Function Call
fixArray(ar, n);
}
// C++ program for above approach
#include <iostream>
using namespace std;
// Function to transform the array
void fixArray(int ar[], int n)
{
int i, j, temp;
// Iterate over the array
for (i = 0; i < n; i++)
{
for (j = 0; j < n; j++)
{
// Check is any ar[j]
// exists such that
// ar[j] is equal to i
if (ar[j] == i) {
temp = ar[j];
ar[j] = ar[i];
ar[i] = temp;
break;
}
}
}
...
// Iterate over array
for (i = 0; i < n; i++)
{
// If not present
if (ar[i] != i)
{
ar[i] = -1;
}
}
...
...
// Print the output
cout << "Array after Rearranging" << endl;
for (i = 0; i < n; i++) {
cout << ar[i] << " ";
}
}
...
...
// Driver Code
int main()
{
int n, ar[] = { -1, -1, 6, 1, 9, 3, 2, -1, 4, -1 };
n = sizeof(ar) / sizeof(ar[0]);
// Function Call
fixArray(ar, n);
}
// Java program for above approach
class GFG{
// Function to transform the array
public static void fixArray(int ar[], int n)
{
int i, j, temp;
// Iterate over the array
for(i = 0; i < n; i++)
{
for(j = 0; j < n; j++)
{
// Check is any ar[j]
// exists such that
// ar[j] is equal to i
if (ar[j] == i)
{
temp = ar[j];
ar[j] = ar[i];
ar[i] = temp;
break;
}
}
}
...
...
// Iterate over array
for(i = 0; i < n; i++)
{
// If not present
if (ar[i] != i)
{
ar[i] = -1;
}
}
...
...
// Print the output
System.out.println("Array after Rearranging");
for(i = 0; i < n; i++)
{
System.out.print(ar[i] + " ");
}
}
...
...
// Driver Code
public static void main(String[] args)
{
int n, ar[] = { -1, -1, 6, 1, 9,
3, 2, -1, 4, -1 };
n = ar.length;
// Function Call
fixArray(ar, n);
}
}
// C# program for above approach
using System;
class GFG {
// Function to transform the array
static void fixArray(int[] ar, int n)
{
int i, j, temp;
// Iterate over the array
for(i = 0; i < n; i++)
{
for(j = 0; j < n; j++)
{
// Check is any ar[j]
// exists such that
// ar[j] is equal to i
if (ar[j] == i)
{
temp = ar[j];
ar[j] = ar[i];
ar[i] = temp;
break;
}
}
}
// Iterate over array
for(i = 0; i < n; i++)
{
// If not present
if (ar[i] != i)
{
ar[i] = -1;
}
}
// Print the output
Console.WriteLine("Array after Rearranging");
for(i = 0; i < n; i++)
{
Console.Write(ar[i] + " ");
}
}
...
static void Main() {
int[] ar = { -1, -1, 6, 1, 9,
3, 2, -1, 4, -1 };
int n = ar.Length;
// Function Call
fixArray(ar, n);
}
}
// This code is contributed by divyeshrabadiya07
S.No. | Searching Algorithm | Sorting Algorithm |
---|---|---|
1. | Searching Algorithms are designed to retrieve an element from any data structure where it is used. | A Sorting Algorithm is used to arranging the data of list or array into some specific order. |
2. | These algorithms are generally classified into two categories i.e. Sequential Search and Interval Search. | There are two different categories in sorting. These are Internal and External Sorting. |
3. | The worst-case time complexity of searching algorithm is O(N). | The worst-case time complexity of many sorting algorithms like Bubble Sort, Insertion Sort, Selection Sort, and Quick Sort is O(N2). |
4. | There is no stable and unstable searching algorithms. | Bubble Sort, Insertion Sort, Merge Sort etc are the stable sorting algorithms whereas Quick Sort, Heap Sort etc are the unstable sorting algorithms. |
5. | The Linear Search and the Binary Search are the examples of Searching Algorithms. | The Bubble Sort, Insertion Sort, Selection Sort, Merge Sort, Quick Sort etc are the examples of Sorting Algorithms. |
1 2 3
4 5 6
7 8 9
4 1 2
7 5 3
8 9 6
For 4*4 matrix
1 2 3 4
5 6 7 8
9 10 11 12
13 14 15 16
Output:
5 1 2 3
9 10 6 4
13 11 7 8
14 15 16 12
// C++ program to rotate a matrix
#include <bits/stdc++.h>
#define R 4
#define C 4
using namespace std;
...
...
// A function to rotate a matrix mat[][] of size R x C.
// Initially, m = R and n = C
void rotatematrix(int m, int n, int mat[R][C])
{
int row = 0, col = 0;
int prev, curr;
/*
row - Starting row index
m - ending row index
col - starting column index
n - ending column index
i - iterator
*/
while (row < m && col < n)
{
if (row + 1 == m || col + 1 == n)
break;
// Store the first element of next row, this
// element will replace first element of current
// row
prev = mat[row + 1][col];
/* Move elements of first row from the remaining rows */
for (int i = col; i < n; i++)
{
curr = mat[row][i];
mat[row][i] = prev;
prev = curr;
}
row++;
/* Move elements of last column from the remaining columns */
for (int i = row; i < m; i++)
{
curr = mat[i][n-1];
mat[i][n-1] = prev;
prev = curr;
}
n--;
/* Move elements of last row from the remaining rows */
if (row < m)
{
for (int i = n-1; i >= col; i--)
{
curr = mat[m-1][i];
mat[m-1][i] = prev;
prev = curr;
}
}
m--;
/* Move elements of first column from the remaining rows */
if (col < n)
{
for (int i = m-1; i >= row; i--)
{
curr = mat[i][col];
mat[i][col] = prev;
prev = curr;
}
}
col++;
}
...
...
// Print rotated matrix
for (int i=0; i<R; i++)
{
for (int j=0; j<C; j++)
cout << mat[i][j] << " ";
cout << endl;
}
}
/* Driver program to test above functions */
int main()
{
// Test Case 1
int a[R][C] = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12},
{13, 14, 15, 16}
};
// Test Case 2
/* int a[R][C] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
*/
rotatematrix(R, C, a);
return 0;
}
// Java program to rotate a matrix
import java.lang.*;
import java.util.*;
class GFG
{
static int R = 4;
static int C = 4;
// A function to rotate a matrix
// mat[][] of size R x C.
// Initially, m = R and n = C
static void rotatematrix(int m,
int n, int mat[][])
{
int row = 0, col = 0;
int prev, curr;
/*
row - Starting row index
m - ending row index
col - starting column index
n - ending column index
i - iterator
*/
while (row < m && col < n)
{
if (row + 1 == m || col + 1 == n)
break;
// Store the first element of next
// row, this element will replace
// first element of current row
prev = mat[row + 1][col];
// Move elements of first row
// from the remaining rows
for (int i = col; i < n; i++)
{
curr = mat[row][i];
mat[row][i] = prev;
prev = curr;
}
row++;
// Move elements of last column
// from the remaining columns
for (int i = row; i < m; i++)
{
curr = mat[i][n-1];
mat[i][n-1] = prev;
prev = curr;
}
n--;
// Move elements of last row
// from the remaining rows
if (row < m)
{
for (int i = n-1; i >= col; i--)
{
curr = mat[m-1][i];
mat[m-1][i] = prev;
prev = curr;
}
}
m--;
// Move elements of first column
// from the remaining rows
if (col < n)
{
for (int i = m-1; i >= row; i--)
{
curr = mat[i][col];
mat[i][col] = prev;
prev = curr;
}
}
col++;
}
// Print rotated matrix
for (int i = 0; i < R; i++)
{
for (int j = 0; j < C; j++)
System.out.print( mat[i][j] + " ");
System.out.print("\n");
}
}
/* Driver program to test above functions */
public static void main(String[] args)
{
// Test Case 1
int a[][] = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12},
{13, 14, 15, 16}
};
// Test Case 2
/* int a[][] = new int {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};*/
rotatematrix(R, C, a);
}
}
// C# program to rotate a matrix
using System;
class GFG {
static int R = 4;
static int C = 4;
// A function to rotate a matrix
// mat[][] of size R x C.
// Initially, m = R and n = C
static void rotatematrix(int m,
int n, int [,]mat)
{
int row = 0, col = 0;
int prev, curr;
/*
row - Starting row index
m - ending row index
col - starting column index
n - ending column index
i - iterator
*/
while (row < m && col < n)
{
if (row + 1 == m || col + 1 == n)
break;
// Store the first element of next
// row, this element will replace
// first element of current row
prev = mat[row + 1, col];
// Move elements of first row
// from the remaining rows
for (int i = col; i < n; i++)
{
curr = mat[row,i];
mat[row, i] = prev;
prev = curr;
}
row++;
// Move elements of last column
// from the remaining columns
for (int i = row; i < m; i++)
{
curr = mat[i,n-1];
mat[i, n-1] = prev;
prev = curr;
}
n--;
// Move elements of last row
// from the remaining rows
if (row < m)
{
for (int i = n-1; i >= col; i--)
{
curr = mat[m-1,i];
mat[m-1,i] = prev;
prev = curr;
}
}
m--;
// Move elements of first column
// from the remaining rows
if (col < n)
{
for (int i = m-1; i >= row; i--)
{
curr = mat[i,col];
mat[i,col] = prev;
prev = curr;
}
}
col++;
}
// Print rotated matrix
for (int i = 0; i < R; i++)
{
for (int j = 0; j < C; j++)
Console.Write( mat[i,j] + " ");
Console.Write("\n");
}
}
/* Driver program to test above functions */
public static void Main()
{
// Test Case 1
int [,]a = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12},
{13, 14, 15, 16}
};
// Test Case 2
/* int a[][] = new int {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};*/
rotatematrix(R, C, a);
}
}
// This code is contributed by nitin mittal.
5 x 6
containing 6
number of non-zero values. This matrix can be represented as shown in the image6
non-zero elements ( those are 9, 8, 4, 2, 5 & 2
) and matrix size is 5 X 6
.5
rows, 6
columns & 6
non-zero values.0, 4, & 9
which indicates the non-zero value 9 is at the 0th-row 4th column in the Sparse matrix.#include<iostream>
using namespace std;
int main()
{
// sparse matrix of class 5x6 with 6 non-zero values
int sparseMatrix[5][6] =
{
{0 , 0 , 0 , 0 , 9, 0 },
{0 , 8 , 0 , 0 , 0, 0 },
{4 , 0 , 0 , 2 , 0, 0 },
{0 , 0 , 0 , 0 , 0, 5 },
{0 , 0 , 2 , 0 , 0, 0 }
};
...
...
// Finding total non-zero values in the sparse matrix
int size = 0;
for (int row = 0; row < 5; row++)
for (int column = 0; column < 6; column++)
if (sparseMatrix[row][column] != 0)
size++;
...
...
// Defining result Matrix
int resultMatrix[3][size];
// Generating result matrix
int k = 0;
for (int row = 0; row < 5; row++)
for (int column = 0; column < 6; column++)
if (sparseMatrix[row][column] != 0)
{
resultMatrix[0][k] = row;
resultMatrix[1][k] = column;
resultMatrix[2][k] = sparseMatrix[row][column];
k++;
}
...
...
// Displaying result matrix
cout<<"Triplet Representation : "<<endl;
for (int row=0; row<3; row++)
{
for (int column = 0; column<size; column++)
cout<<resultMatrix[row][column]<<" ";
cout<<endl;
}
return 0;
}
H0, H1,..., H5
indicates the header nodes which are used to represent indexes.
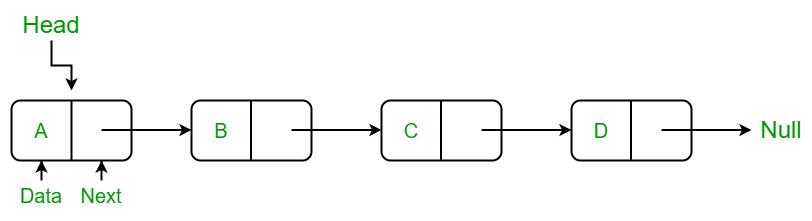


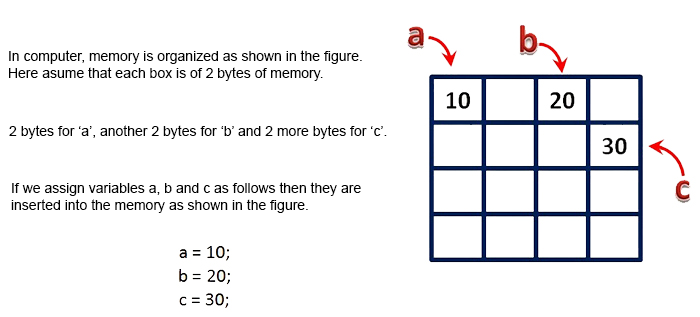


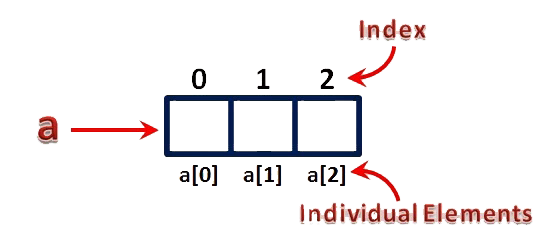
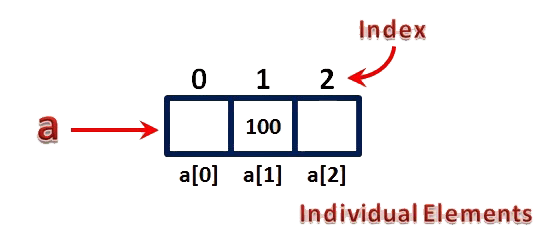

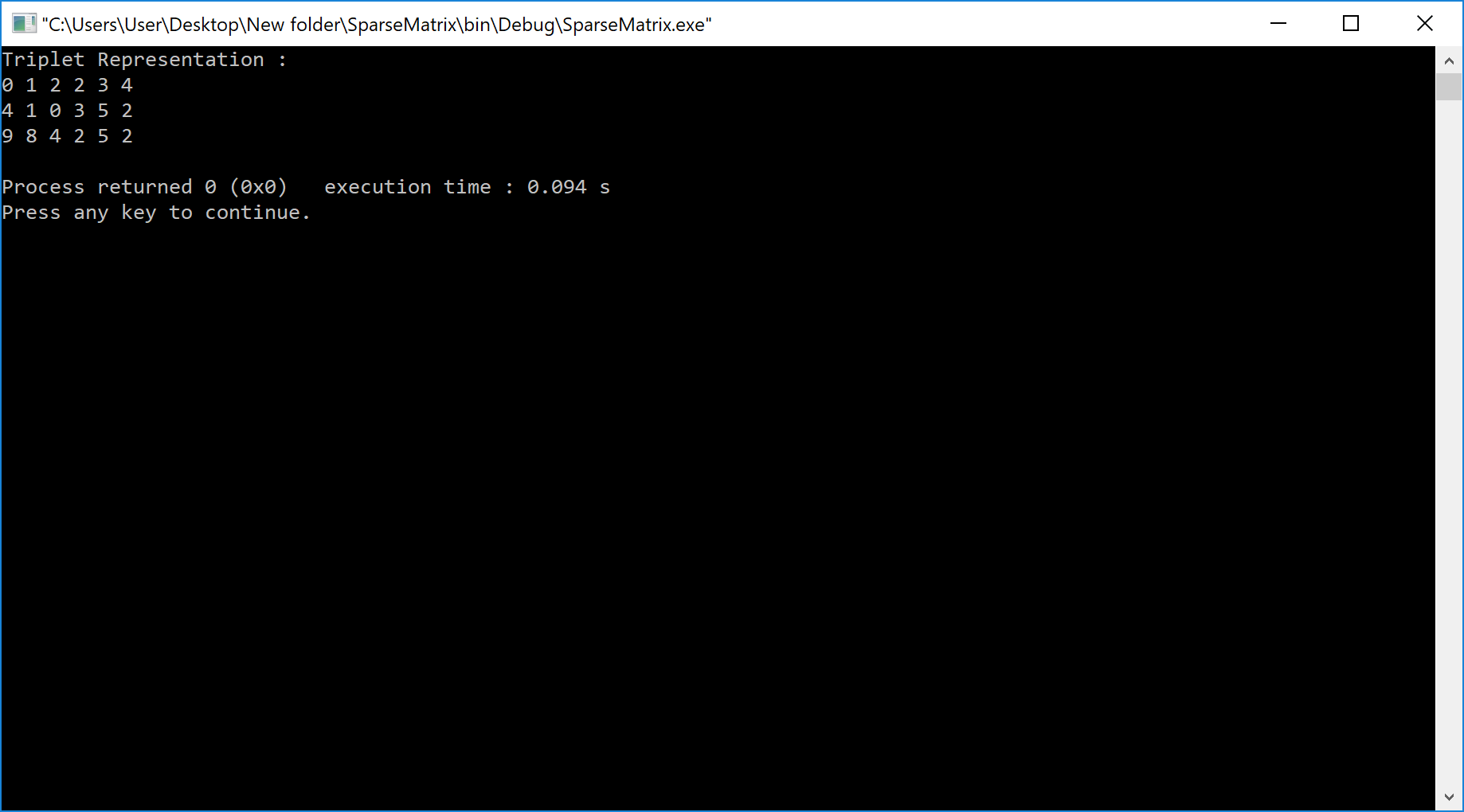

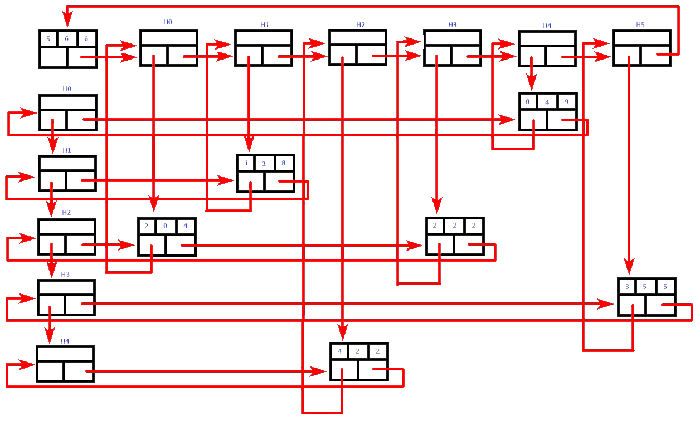